So, I wanted to try to get fancy with my dates, namely wanting to make it display as either "MMMM D, YYYY", "MMMM YYYY" or "YYYY". In my setup, <Year> is stored as 4-digit value and the month and year are stored in another tag (<DateCustom>) as "month.day". I used <DateCustom> for creating folder names and sorting under Mediamonkey. Using the virtualtag <DateTest> below, I get the following result: YYYY, 6-digit YYYYMM, or 8-digit YYYYMMDD. The reason why there's a 6-digit and 8-digit result is due to $Date returning a null result when faced with "00" (Y2K holdover?). So, here's the virtualtags:
DateTest: $IsNull(<DateCustom>,<Year (yyyy)>,$If(<DateCustom>=00.00,<Year (yyyy)>,$If($Right(<DateCustom>,2)=00,<Year (yyyy)>$Left(<DateCustom>,2),<Year (yyyy)>$Replace(<DateCustom>,.,))))
For $Date, I used the following:
* 8-digit YYYYMMDD: $Date($Left(<DateTest>,4)/$Left($Right(<DateTest>,4),2)/$Right(<DateTest>,2),MMMM d"," yyyy)
* 6-digit YYYYMM: $Date($Left(<DateTest>,4)/$Right(<DateTest>,2),MMMM yyyy)
Everything works so far, but the difficulty is finding a way to have the parser recognize the 6-digit and 8-digit strings and apply the appropriate function. Ideally, I would use regex (^[\d]{6}$ or ^[\d]{8}$) to sniff out those values (basically a search for 6- or 8-length digits), but it would only return T or F as values. Well, here comes $If(value,false,true) function to the rescue!
Here is how I manage to get the date to show up as either "YYYY", "MMMM YYYY" or "MMMM d, YYYY" as seen in the attached pic below:
DateFormat: $IsNull(<DateCustom>,<Year (yyyy)>,$If($IsMatch(<DateTest>,"^[\d]{6}$")=T, $Date($Left(<DateTest>,4)/$Right(<DateTest>,2),MMMM yyyy), $Date($Left(<DateTest>,4)/$Left($Right(<DateTest>,4),2)/$Right(<DateTest>,2),MMMM d"," yyyy)))
I hope someone here finds this useful.
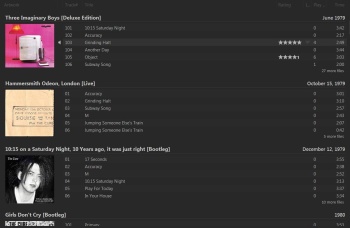
Here's an extra bit of code if someone simply wants M/d/YYYY (derived from <DateCustom> = month.day, including "month.00" [i.e. 07.00])
ReleaseDate: $IsNull(<DateCustom>,<Year (yyyy)>,$If(<DateCustom>=00.00,<Year (yyyy)>,$If($Right(<DateCustom>,2)=00,$If($Left(<DateCustom>,1)=0,$Right($Left(<DateCustom>,2),1)/<Year (yyyy)>,$Left(<DateCustom>,2)/<Year (yyyy)>),$Replace($Replace(<DateCustom>,0,),.,/)/<Year (yyyy)>)))